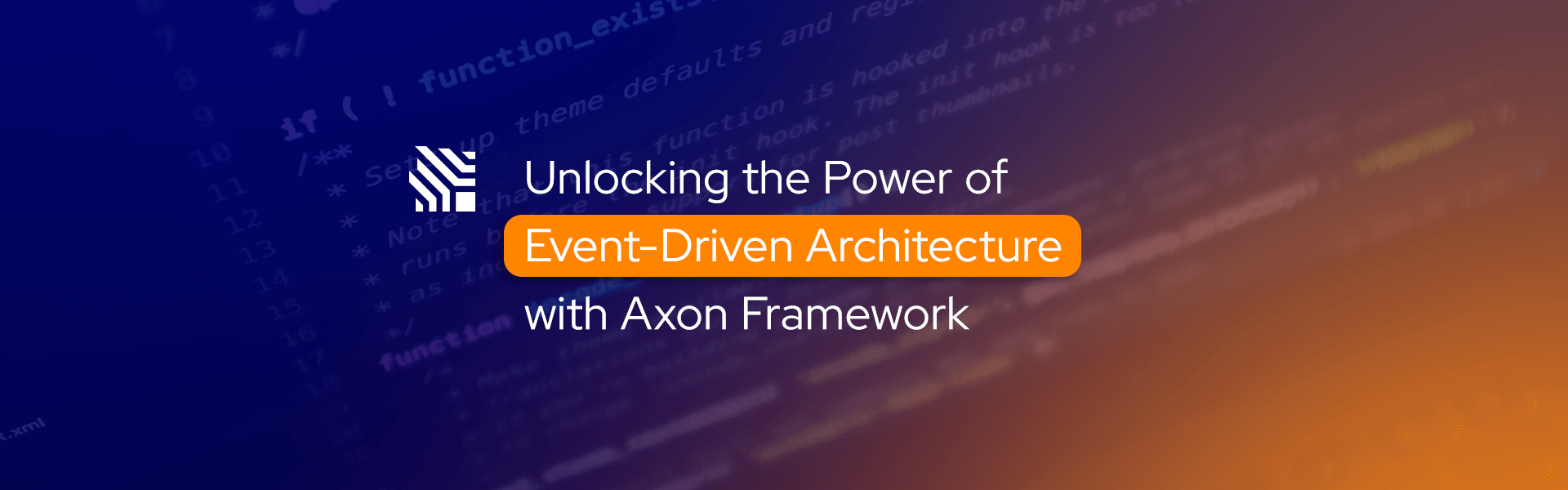
Unlocking the Power of Event-Driven Architecture with Axon Framework
Discover the power of event-driven architecture and learn how Axon Framework simplifies the implementation of scalable and maintainable systems. This comprehensive guide explores the fundamental patterns, benefits, and best practices of building event-driven microservices using Axon Framework.
15 min read
In this Article:
- Introduction to event-driven architecture and its benefits
- Overview of Axon Framework and its key features
- Fundamental patterns of event-driven architecture
- Deploying and managing event-driven systems with Axon Framework
- Conclusion and getting started with Axon Framework
Introduction
In today’s fast-paced and ever-evolving software development landscape, building systems that are scalable, maintainable, and adaptable to change is more critical than ever. Event-driven architecture (EDA) has emerged as a powerful paradigm for designing and building such systems. EDA focuses on the production, detection, consumption, and reaction to events, enabling loose coupling, flexibility, and scalability.
In this blog post, we’ll explore the fundamental concepts and patterns of event-driven architecture and how the Axon Framework, a leading open-source framework for building event-driven microservices, can help you unlock the full potential of EDA. We’ll dive into the benefits of EDA, discuss key patterns such as event sourcing and Command Query Responsibility Segregation (CQRS), and showcase how Axon Framework simplifies the implementation of these patterns.
Whether you’re building microservices, cloud-native applications, or distributed systems, understanding event-driven architecture and leveraging frameworks like Axon can empower you to create systems that are resilient, scalable, and capable of handling the demands of modern software development.
Why Event-Driven Architecture Matters
In traditional architectures, components are often tightly coupled, leading to systems that are difficult to scale, maintain, and evolve. Event-driven architecture addresses these challenges by promoting loose coupling and asynchronous communication between components. By focusing on events as the core building blocks, EDA enables systems to react to changes in real-time, scale independently, and adapt to new requirements more easily.
Some key benefits of event-driven architecture include:
- Scalability: EDA allows components to scale independently based on their specific needs, as they communicate through asynchronous events rather than direct calls.
- Flexibility: With EDA, introducing new functionality or modifying existing components becomes easier, as changes can be made without impacting the entire system.
- Maintainability: Loose coupling and clear separation of concerns in EDA lead to systems that are easier to understand, test, and maintain over time.
- Resilience: EDA promotes fault tolerance and resilience by enabling components to react to and recover from failures independently.
Introducing Axon Framework
Axon Framework is a powerful open-source framework that simplifies the implementation of event-driven architectures and microservices. It provides a set of abstractions and building blocks that align with the principles of Domain-Driven Design (DDD), Command Query Responsibility Segregation (CQRS), and Event Sourcing.
Some of the key features and benefits of Axon Framework include:
- Event Sourcing: Axon Framework provides a robust event sourcing mechanism, allowing you to persist the state of your system as a sequence of events, enabling powerful capabilities like event replay and auditing.
- CQRS: Axon Framework supports the separation of command and query responsibilities, enabling you to optimize read and write operations independently and scale them separately.
- Sagas: Axon Framework includes a powerful implementation of sagas, allowing you to model complex, long-running business processes as a series of events and reactions.
- Event-Driven Communication: With Axon Framework, you can easily implement event-driven communication between components using the built-in event bus and messaging abstractions.
- Scalability and Performance: Axon Framework is designed with scalability and performance in mind, providing features like event stream partitioning and distributed event processing.
In the following sections, we’ll explore the fundamental patterns and concepts of event-driven architecture and how Axon Framework helps you implement them effectively. Let’s dive in and unlock the power of EDA with Axon Framework!
Event-Driven Architecture Patterns
Event-driven architecture (EDA) is a software architecture paradigm that promotes the production, detection, consumption of, and reaction to events. This chapter will explore the fundamental patterns in EDA, including events, event producers, event consumers, and the event store. We’ll also showcase examples of how these concepts are implemented using the Axon Framework.
Events
An event is an immutable record of something that has happened in the system. It captures information about a state change or an action that occurred at a specific point in time. Events are typically expressed in the past tense, such as “OrderPlaced”, “PaymentConfirmed”, etc.
Here’s an example of an event definition in Axon:
public class OrderPlaced {
private final String orderId;
private final List<OrderLine> orderLines;
private final BigDecimal totalAmount;
// constructor, getters, equals/hashCode
}
Events should be as specific as possible and contain only the information necessary to describe what happened. This makes them easy to understand and process by other components in the system.
Event Producers
An event producer is a component that generates events in response to state changes or user actions. For example, it could be a domain service that creates an “OrderPlaced” event after a customer successfully places an order.
In Axon Framework, event producers are typically represented by aggregates. An aggregate is a domain object that encapsulates state and business logic related to a specific business entity. When the state of an aggregate changes, it emits events describing that change.
Here’s an example of an Order
aggregate in Axon:
@Aggregate
public class Order {
@AggregateIdentifier
private String orderId;
private List<OrderLine> orderLines;
private BigDecimal totalAmount;
private OrderStatus status;
@CommandHandler
public Order(PlaceOrder command) {
// validate command
apply(new OrderPlaced(command.getOrderId(),
command.getOrderLines(),
command.getTotalAmount()));
}
@EventSourcingHandler
public void on(OrderPlaced event) {
this.orderId = event.getOrderId();
this.orderLines = event.getOrderLines();
this.totalAmount = event.getTotalAmount();
this.status = OrderStatus.PLACED;
}
// other methods
}
In this example, the @CommandHandler
method handles the PlaceOrder
command and generates an OrderPlaced
event using the apply()
method. The @EventSourcingHandler
method updates the aggregate’s state based on the emitted event.
Event Consumers
An event consumer is a component that listens for specific event types and reacts to them by performing appropriate actions. For example, it could be a notification service that sends an email to the customer when an “OrderShipped” event is received.
In Axon Framework, event consumers are implemented as event handlers. An event handler is a class containing one or more methods annotated with @EventHandler
that process specific event types.
Here’s an example of an event handler in Axon:
@Component
public class CustomerNotificationEventHandler {
private final EmailService emailService;
public CustomerNotificationEventHandler(EmailService emailService) {
this.emailService = emailService;
}
@EventHandler
public void on(OrderShipped event) {
String emailAddress = event.getEmailAddress();
String emailContent = "Your order " + event.getOrderId() + " has been shipped.";
emailService.sendEmail(emailAddress, "Order Shipped", emailContent);
}
}
In this example, the on()
method is invoked whenever an OrderShipped
event occurs in the system. The handler uses the EmailService
to send an email notification to the customer informing them that their order has been shipped.
Event Store
An event store is a specialized database optimized for storing streams of events generated by the system. Unlike traditional databases that store the current state, an event store persists all the events that led to the current state. This allows the system’s state to be reconstructed at any point in time by replaying the events.
Axon Framework provides its own implementation of an event store called AxonServerEventStore, which stores events in a dedicated Axon Server database. Alternatively, Axon can be configured to work with other databases such as MongoDB, PostgreSQL, or Apache Kafka.
Here’s an example of configuring an event store in Axon:
@Configuration
public class AxonConfig {
@Bean
public EventStore eventStore() {
return AxonServerEventStore.builder()
.configuration(AxonServerConfiguration.builder()
.servers("localhost:8124")
.build()
).build();
}
}
In this example, we create an instance of AxonServerEventStore
that connects to a local Axon Server instance running on port 8124.
Event Processing and State Management
In an event-driven architecture, event processing and state management are crucial aspects that ensure the system maintains a consistent and accurate state. This chapter will delve into event sourcing, event projections, materialized views, sagas, and event-based state management. We’ll explore how these concepts are implemented using the Axon Framework and provide practical examples to illustrate their usage.
Event Sourcing
Event sourcing is a design pattern that involves persisting the state of a system as a sequence of events. Instead of storing the current state directly, event sourcing stores all the events that have happened in the system. The current state can be derived by replaying the events from the beginning of time.
In Axon Framework, event sourcing is implemented using aggregates. An aggregate is an entity that encapsulates state and behavior, and it is responsible for maintaining its own state consistency. When an aggregate’s state changes, it emits events that represent those changes.
Here’s an example of an event-sourced BankAccount
aggregate in Axon:
@Aggregate
public class BankAccount {
@AggregateIdentifier
private String accountId;
private BigDecimal balance;
@CommandHandler
public BankAccount(OpenBankAccount command) {
apply(new BankAccountOpened(command.getAccountId(), command.getInitialBalance()));
}
@EventSourcingHandler
public void on(BankAccountOpened event) {
this.accountId = event.getAccountId();
this.balance = event.getInitialBalance();
}
@CommandHandler
public void handle(DepositMoney command) {
apply(new MoneyDeposited(command.getAccountId(), command.getAmount()));
}
@EventSourcingHandler
public void on(MoneyDeposited event) {
this.balance = this.balance.add(event.getAmount());
}
// other methods
}
In this example, the BankAccount
aggregate handles commands such as OpenBankAccount
and DepositMoney
. When these commands are handled, the aggregate emits corresponding events (BankAccountOpened
and MoneyDeposited
) using the apply()
method. The @EventSourcingHandler
methods update the aggregate’s state based on the emitted events.
Snapshots
Snapshot is a representation of the current state of an aggregate at a specific point in time. Instead of replaying all the events from the beginning, we can take a snapshot of the aggregate’s state at regular intervals or based on certain conditions. When reconstructing the state, we can start from the most recent snapshot and replay only the events that occurred after that snapshot.
Axon Framework provides built-in support for creating and managing snapshots. You can configure Axon to automatically create snapshots based on the number of events or a specific trigger.
Here’s an example of how to configure Axon to take snapshots every 100 events:
@Bean
public SnapshotTriggerDefinition snapshotTriggerDefinition(Snapshotter snapshotter) {
return new EventCountSnapshotTriggerDefinition(snapshotter, 100);
}
In this example, we define a SnapshotTriggerDefinition
bean that uses the EventCountSnapshotTriggerDefinition
to trigger snapshots every 100 events. The Snapshotter
is responsible for serializing and deserializing the snapshot data.
When using snapshots, Axon will automatically retrieve the most recent snapshot and replay the events that occurred after that snapshot when reconstructing the aggregate state. This can significantly improve performance and reduce the time required to load an aggregate.
It’s important to note that snapshots are an optimization technique and should be used judiciously. The frequency of taking snapshots depends on factors such as the event volume, the complexity of the aggregate, and the acceptable recovery time.
Event Projections and Materialized Views
Event projections and materialized views are mechanisms for deriving read-optimized representations of the system’s state from the event stream. They allow efficient querying and presentation of data without the need to replay all events each time.
In Axon Framework, event projections are implemented using event handlers that listen for specific events and update a read-optimized data store accordingly. These projections can be stored in various databases or search engines, such as MongoDB, Elasticsearch, or Apache Cassandra.
Here’s an example of an event projection that maintains a materialized view of bank account balances:
@Component
public class BankAccountProjection {
private final MongoTemplate mongoTemplate;
public BankAccountProjection(MongoTemplate mongoTemplate) {
this.mongoTemplate = mongoTemplate;
}
@EventHandler
public void on(BankAccountOpened event) {
BankAccountView view = new BankAccountView();
view.setAccountId(event.getAccountId());
view.setBalance(event.getInitialBalance());
mongoTemplate.save(view);
}
@EventHandler
public void on(MoneyDeposited event) {
BankAccountView view = mongoTemplate.findById(event.getAccountId(), BankAccountView.class);
view.setBalance(view.getBalance().add(event.getAmount()));
mongoTemplate.save(view);
}
// other methods
}
In this example, the BankAccountProjection
listens for BankAccountOpened
and MoneyDeposited
events and updates a BankAccountView
in MongoDB. The BankAccountView
represents a read-optimized view of the bank account state, allowing efficient querying of account balances.
Sagas
Sagas are a pattern for managing long-running business transactions that span multiple aggregates or bounded contexts. They coordinate the execution of multiple steps in a business process, ensuring that each step is executed successfully or compensating actions are taken in case of failures.
In Axon Framework, sagas are implemented as classes annotated with @Saga
. They react to events and dispatch commands to trigger the next steps in the business process.
Here’s an example of a saga that coordinates the process of creating an order and reserving inventory:
@Saga
public class CreateOrderSaga {
@Autowired
private transient CommandGateway commandGateway;
private String orderId;
private String customerId;
private List<OrderLine> orderLines;
@StartSaga
@SagaEventHandler(associationProperty = "orderId")
public void handle(OrderCreatedEvent event) {
this.orderId = event.getOrderId();
this.customerId = event.getCustomerId();
this.orderLines = event.getOrderLines();
// Reserve inventory for each order line
for (OrderLine orderLine : orderLines) {
commandGateway.send(new ReserveInventoryCommand(orderLine.getProductId(), orderLine.getQuantity()));
}
}
@SagaEventHandler(associationProperty = "orderId")
public void handle(InventoryReservedEvent event) {
// Inventory reserved successfully, proceed with order confirmation
commandGateway.send(new ConfirmOrderCommand(orderId));
}
@EndSaga
@SagaEventHandler(associationProperty = "orderId")
public void handle(OrderConfirmedEvent event) {
// Order confirmed, saga ends
}
// other methods
}
In this example, the CreateOrderSaga
starts when an OrderCreatedEvent
is received. It reserves inventory for each order line by sending ReserveInventoryCommand
commands. When all inventory is successfully reserved (InventoryReservedEvent
), the saga proceeds with order confirmation by sending a ConfirmOrderCommand
. Finally, when the OrderConfirmedEvent
is received, the saga ends.
Event-Based Communication
Event-based communication is a key aspect of event-driven architecture that enables loose coupling and asynchronous communication between components. In this chapter, we’ll explore the concepts of domain events, integration events, event publishing, event subscribing, event buses, and event-driven microservices. We’ll also demonstrate how these concepts are implemented using the Axon Framework.
Domain Events vs Integration Events
Events can be classified into two main categories: domain events and integration events.
Domain events represent important occurrences within a specific bounded context or domain. They are typically used for communication within the same microservice or bounded context. Examples of domain events include OrderPlaced
, PaymentProcessed
, and InventoryReserved
.
Integration events, on the other hand, are used for communication between different microservices or bounded contexts. They represent significant occurrences that are of interest to other parts of the system. Examples of integration events include OrderConfirmed
, PaymentApproved
, and InventoryAllocated
.
Here’s a diagram illustrating the distinction between domain events and integration events:
Event Publishing and Subscribing
Event publishing and subscribing are the mechanisms by which components communicate using events. Publishers emit events to notify interested subscribers about important occurrences, while subscribers listen for specific events and react accordingly.
In Axon Framework, event publishing is done using the EventBus
abstraction. The EventBus
is responsible for distributing events to registered subscribers.
Here’s an example of publishing an event using Axon’s EventBus
:
@Autowired
private EventBus eventBus;
public void placeOrder(PlaceOrderCommand command) {
// Perform business logic
// ...
// Publish an event
eventBus.publish(new OrderPlacedEvent(command.getOrderId(), command.getCustomerId(), command.getOrderLines()));
}
Subscribing to events is done by implementing event handlers and registering them with the EventBus
. Event handlers are methods annotated with @EventHandler
that specify the event types they are interested in.
Here’s an example of subscribing to an event in Axon:
@Component
public class OrderNotificationHandler {
@EventHandler
public void on(OrderPlacedEvent event) {
// Send notification to the customer
// ...
}
}
Event Buses and Event-Driven Microservices
Event buses play a central role in event-driven microservices architecture. They act as the communication backbone, allowing microservices to exchange events asynchronously.
Axon Framework provides several implementations of the EventBus
interface, including:
SimpleEventBus
: An in-memory event bus suitable for single-instance applications.AxonServerEventStore
: An event bus that uses Axon Server as the event store and message broker.SpringCloudEventBus
: An event bus that integrates with Spring Cloud Stream for distributed event publishing and subscribing.
Here’s an example of configuring Axon to use Axon Server as the event bus:
@Configuration
public class AxonConfig {
@Bean
public EventBus eventBus() {
return AxonServerEventStore.builder()
.configuration(AxonServerConfiguration.builder()
.servers("localhost:8124")
.build()
).build();
}
}
In an event-driven microservices architecture, each microservice typically has its own event bus for publishing and subscribing to events within its bounded context. Integration events are used for communication between microservices, often via a message broker like Apache Kafka or RabbitMQ.
Here’s a diagram illustrating event-driven communication in a microservices architecture:
Compositional Patterns
Compositional patterns in event-driven architecture focus on designing systems by composing smaller, reusable components or services. These patterns enable building complex systems that are maintainable, scalable, and adaptable to changing requirements. In this chapter, we’ll explore several compositional patterns, including event-driven business processes, process orchestration, Command Query Responsibility Segregation (CQRS), and event sourcing. We’ll also demonstrate how these patterns can be implemented using the Axon Framework.
Business Processes as Event Streams
In event-driven architecture, business processes can be modeled as a series of events that represent the state changes and activities within the process. Each event captures a significant moment or decision point in the process, and the sequence of events forms an event stream that represents the entire process.
By modeling business processes as event streams, we can achieve a high level of flexibility and modularity. Each event can be handled by one or more event handlers, allowing for loose coupling between the process steps. This enables easier modification and extension of the process without impacting other parts of the system.
Here’s an example of modeling an order fulfillment process as an event stream:
Process Orchestration with Events
Process orchestration involves coordinating and managing the execution of multiple services or components to achieve a specific business goal. In event-driven architecture, process orchestration can be achieved using events to trigger and coordinate the activities of different services.
One way to implement process orchestration is by using a saga, which is a pattern for managing long-running transactions or processes. A saga consists of a series of steps, where each step is triggered by an event and can emit one or more events to trigger the next steps in the process.
Here’s an example of a saga that orchestrates the order fulfillment process:
@Saga
public class OrderFulfillmentSaga {
@StartSaga
@SagaEventHandler(associationProperty = "orderId")
public void handle(OrderPlacedEvent event) {
// Start the order fulfillment process
// ...
}
@SagaEventHandler(associationProperty = "orderId")
public void handle(PaymentProcessedEvent event) {
// Proceed with inventory reservation
// ...
}
@SagaEventHandler(associationProperty = "orderId")
public void handle(InventoryReservedEvent event) {
// Proceed with order shipping
// ...
}
@EndSaga
@SagaEventHandler(associationProperty = "orderId")
public void handle(OrderDeliveredEvent event) {
// Complete the order fulfillment process
// ...
}
}
In this example, the OrderFulfillmentSaga
orchestrates the order fulfillment process by handling events and triggering the appropriate actions at each step of the process.
CQRS and Event Sourcing
Command Query Responsibility Segregation (CQRS) is a pattern that separates the write operations (commands) from the read operations (queries) in a system. CQRS allows optimizing the read and write paths independently, enabling better performance, scalability, and maintainability.
Event sourcing is often used in conjunction with CQRS to persist the state of the system as a sequence of events. Instead of storing the current state, event sourcing stores all the events that have occurred in the system. The current state can be reconstructed by replaying the events.
Here’s an example of implementing CQRS and event sourcing using Axon Framework:
// Command Model
@Aggregate
public class Order {
@AggregateIdentifier
private String orderId;
private OrderStatus status;
@CommandHandler
public Order(PlaceOrderCommand command) {
// Validate and handle the command
// ...
apply(new OrderPlacedEvent(command.getOrderId(), command.getCustomerId(), command.getOrderLines()));
}
@EventSourcingHandler
public void on(OrderPlacedEvent event) {
this.orderId = event.getOrderId();
this.status = OrderStatus.PLACED;
}
}
// Query Model
@Component
public class OrderProjection {
@EventHandler
public void on(OrderPlacedEvent event) {
// Update the read model
// ...
}
}
In this example, the Order
aggregate represents the command model and handles the PlaceOrderCommand
by emitting an OrderPlacedEvent
. The OrderProjection
represents the query model and handles the OrderPlacedEvent
to update the read model.
Deploying and Managing Event-Driven Systems
Deploying and managing event-driven systems requires careful consideration of various aspects, such as event stream partitioning, event replay, monitoring, and handling of duplicate events. In this chapter, we’ll explore these topics and provide examples of how to address them using the Axon Framework.
Event Stream Partitioning
Event stream partitioning is a technique used to scale event-driven systems by distributing the processing of events across multiple instances or nodes. Partitioning allows for parallel processing of events and improves the overall throughput and performance of the system.
In Axon Framework, event stream partitioning can be achieved using the SequencingPolicy
abstraction. The SequencingPolicy
determines how events are assigned to a specific processing group or partition.
Here’s an example of configuring event stream partitioning in Axon:
@Bean
public SequencingPolicy sequencingPolicy() {
return new SimpleSequencingPolicy();
}
In this example, we configure a SimpleSequencingPolicy
, which assigns events to a processing group based on the aggregate identifier. This ensures that events for the same aggregate are processed in a sequential manner.
Event Replay and Idempotence
Event replay is the ability to replay events from a specific point in time to reconstruct the state of the system or to update projections. Event replay is useful for various scenarios, such as fixing bugs, updating read models, or recovering from failures.
When replaying events, it’s crucial to ensure idempotence, which means that the processing of an event multiple times should produce the same result as processing it once. Idempotence is necessary to handle duplicate events that may occur due to network issues or retries.
Axon Framework provides support for event replay through the TrackingEventProcessor
. The TrackingEventProcessor
keeps track of the last processed event and allows replaying events from a specific position.
Here’s an example of configuring event replay in Axon:
@Bean
public TrackingEventProcessor trackingEventProcessor(EventHandlingConfiguration eventHandlingConfiguration, EventBus eventBus) {
return TrackingEventProcessor.builder()
.name("order-projection")
.eventHandlerInvoker(eventHandlingConfiguration.eventHandlerInvoker())
.messageSource(eventBus)
.build();
}
In this example, we configure a TrackingEventProcessor
named “order-projection” that replays events from the event bus and invokes the corresponding event handlers.
To ensure idempotence, you can use the @IdempotentHandler
annotation on your event handlers. This annotation indicates that the event handler is idempotent and can safely handle duplicate events.
@IdempotentHandler
@EventHandler
public void on(OrderPlacedEvent event) {
// Update the read model
// ...
}
Monitoring and Observability
Monitoring and observability are critical aspects of managing event-driven systems. They provide insights into the system’s behavior, performance, and health, enabling proactive identification and resolution of issues.
Axon Framework integrates with various monitoring and observability tools, such as Micrometer and Prometheus, to provide metrics and tracing capabilities.
Here’s an example of configuring monitoring in Axon using Micrometer:
@Bean
public MeterRegistry meterRegistry() {
return new PrometheusMeterRegistry();
}
In this example, we configure a PrometheusMeterRegistry
to collect and expose metrics from Axon Framework.
Additionally, Axon Framework provides tracing capabilities through the MessageTrackingDispatchInterceptor
. This interceptor captures trace information for each message processed by Axon, allowing for end-to-end tracing of event-driven flows.
Conclusion
Event-driven architecture, powered by frameworks like Axon, offers a compelling approach to building modern, scalable, and resilient systems. By embracing events as the core building blocks and leveraging patterns such as event sourcing, CQRS, and sagas, developers can create systems that are highly adaptable, maintainable, and scalable. Axon Framework provides a comprehensive set of tools and abstractions that simplify the implementation of event-driven systems, making it easier to build and evolve complex applications over time. Whether you’re building microservices, cloud-native applications, or distributed systems, Axon Framework offers a solid foundation for creating event-driven architectures. We hope this blog post has provided you with valuable insights into event-driven architecture and the capabilities of Axon Framework. We encourage you to explore the resources mentioned above and start building your own event-driven systems using Axon Framework. Happy coding, and may your events flow seamlessly!
Key Takeaways
- Event-driven architecture enables building loosely coupled, scalable, and maintainable systems.
- Axon Framework provides a comprehensive set of abstractions and tools for implementing event-driven systems, including event sourcing, CQRS, sagas, and event-based communication.
- Event processing and state management patterns, such as event sourcing and materialized views, allow for building highly adaptable and scalable systems.
- Event-based communication enables loose coupling and asynchronous communication between components, promoting scalability and flexibility.
- Compositional patterns, such as event-driven process orchestration and CQRS, enable building modular and maintainable systems.
- Deploying and managing event-driven systems requires careful consideration of event stream partitioning, event replay, idempotence, monitoring, and observability.
Getting Started with Axon Framework
If you’re interested in building event-driven systems using Axon Framework, here are some resources to get you started:
- Axon Framework Documentation: The official documentation provides comprehensive guides, tutorials, and reference material for using Axon Framework.
- Axon Framework Samples: The Axon Framework GitHub repository contains various code samples and example projects showcasing different aspects of event-driven systems.
- Axon Framework Community: The Axon Framework community forum is a great place to ask questions, seek guidance, and engage with other developers using Axon Framework.